Introduction
We use Python bool types True and False often in our program. Do you want to know how to works behind the scenes? In this article, you will get a thorough understanding of Python bool type, how it works, and how to effectively use it in your Python application. Without further adieu, let’s get started.
Bool Type
In Python, the bool data type is used to represent boolean values —True and False.
As you know everything is an object in Python. That means integers, floats, strings, lists, tuples, etc. are all objects of their respective class. For example, integers are objects ‘int’ class, floats are the object of ‘float’ class, lists are objects of ‘list’ class.
Similarly, boolean values True and False are also the objects of the class bool. The built-in type() function returns the data-type. As you can see from the below code, True and False are bool types, meaning objects of the class bool.
>>> print(type(True))
>>> print(type(False))
Boolean values are commonly used when comparing the values and also in if and while statements.
>>> 10 > 100
False
>>> 10 == 10
True
>>> x, y = 10, 11
>>> if x == y:
... print("x is equal to y")
... else:
... print("x is not equal to y")
...
x is not equal to y
The bool data type is a subclass of int. As a result, it exhibits all the properties of int. In other words, we can carry out mathematical operations and other special operations on boolean values. Also note that True and False behave like the integers 1 and 0, respectively.
>>> int(True)
1
>>> int(False)
0
>>> True == 1
True
>>> False == 0
True
>>> True > 0
True
>>> False < 1
True
>>> True + True + True
3
>>> True + True + 1
3
>>> True * 3 + False
3
>>> False + False + 3
3
The knowledge about truth value and how it is evaluated is crucial for understanding bool type and its operations.
Truth Value
Every object in Python has its truth value. These truth values are useful while evaluating boolean expressions. But the question is when an object returns True or False?
In general, all non-zero numbers and non-empty sequence types & collections return True. Similarly, all numeric numbers with value zero and empty sequences & collections including None return False.
In short, almost all of the objects return True except for a handful of objects listed below that return False.
- None and False.
- Numbers with value zero: 0(int), 0.0(float), 0j(complex), Decimal(0)(Decimal), Fraction(0,1)(Fraction)
- Empty sequences types and collections: “”(string), ()(tuple), [](list), {}(dictionary), set()(set), range(0).
- Custom classes that implement __bool__() or __len__() methods that return False or zero.
How truth value is evaluated internally?
Here is the definition from the official documentation — “An object returns True unless it’s class implements __bool__() method that returns False or __len__() method that returns zero.”
Let’s try to understand this with two examples.
Example 1: In the below example, x is an integer object referencing the value 10. During the execution of if x, Python knows that it has to evaluate x for its truth value. Here x is an object of int class and int class implements __bool__() method. Since the 10 is a non-zero value, __bool__() returns True, and prints “I am inside if”.
What is __bool__()? It is one of the special methods or magic functions that is called during truth value testing. It is also called when the built-in function bool() is invoked. This method returns True or False as per the rules mentioned earlier.
x = 10
if x:
print('I am inside if')
else:
print('I am inside else')
Example 2: In the below example, y is an empty list. During the execution of if y, y is evaluated for its truth value by Python. Here y is an object of list class and list class implements __len__() method. As defined earlier, for empty sequence types such as lists, __len__() returns False and prints ‘I am inside else’.
y = []
if y:
print('I am inside if')
else:
print('I am inside else')
What is __len__()? It is also one of the special methods or magic functions that is called when the built-in function len() is invoked. This method returns True or False when the object is an empty sequence type or collection.
So, in a nutshell, during truth value evaluation, the __bool__() method is called first on the object. If __bool__() method is not defined then __len__() method is called. If both are not defined for the class then it returns True for all its instances.
The bool() method
The bool method bool() takes in an argument x and returns its truth value — True or False. Some classes implement __bool__() and some __len__(). So instead of checking them individually, you can use bool(). When we use bool, Python automatically calls __bool__() method first. If __bool__() is not defined then __len__() method is called. If both are not defined for the class then it returns True for all its instances.
>>> x = [] #empty list
>>> y = 10
>>> x.__len__()
0
>>> y.__bool__()
True
>>> x.__bool__()
Traceback (most recent call last):
File "", line 1, in
AttributeError: 'list' object has no attribute '__bool__'
>>> y.__len__()
Traceback (most recent call last):
File "", line 1, in
AttributeError: 'int' object has no attribute '__len__'
>>> bool(x)
False
>>> bool(y)
True
>>> bool([1,2,3])
True
>>> bool(None)
False
>>> bool(0), bool(0.0), bool(Decimal('0')), bool(None), bool(False)
(False, False, False, False, False)
Boolean operators and precedence
The boolean operators are: and, or, not.
The precedence of boolean operators along with other operators is shown below. The below list shows increasing order of precedence i.e. lowest precedence is at the top and the highest precedence is at the bottom. As you can see from the list the precedence of boolean operators is — not, and, or (not has higher precedence and or has the lowest precedence).
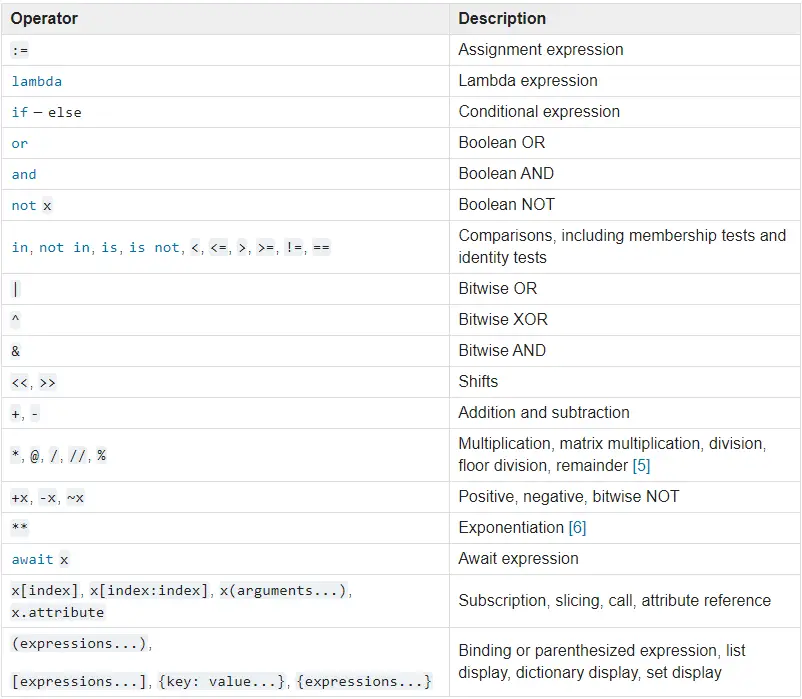
Short-circuiting
In Python, boolean operators and & or works differently using the technique called short-circuiting. The below tables summarizes short-circuiting operator for and and or. Note that or operator along with many other operators returns either 0 or False for false and 1 or True for true.

Let’s look into some examples to better understand the short-circuiting operations. As you can see boolean operators and and or don’t return True or False. Instead, both return either first operand (x) or second operand (y).
>>> x, y, z = 10, 20, 0
>>> not z
True
>>> not x
False
>>> x and y
20
>>> x and z
0
>>> x or y
10
>>> x or z
10
Conclusion
In this article, you understood boolean operators in great detail. You got to know about what happens behind the scenes during truth value testing of python objects. You also learned about the bool() method, operator precedence, and short-circuit operation.