Introduction
In this article, we will go through one of the memory management techniques in Python called Reference Counting.
In Python, all objects and data structures are stored in the private heap and are managed by Python Memory Manager internally. The goal of the memory manager is to ensure that enough space is available in the private heap for memory allocation. This is done by deallocating the objects that are not currently being referenced (used). As developers, we don’t have to worry about it as it will be handled automatically by Python.
Reference Counting
Reference counting is one of the memory management technique in which the objects are deallocated when there is no reference to them in a program. Let’s try to understand with examples.
Variables in Python are just the references to the objects in the memory. In the below example, when Python executes var1 = [10, 20]
, the integer object [10, 20]
is stored in some memory location 0x20bfa819cc8
, and var1
is only the reference to that object in the memory. This means var1
doesn’t contain the value [10, 20]
but references to the address 0x20bfa819cc8
in the memory.
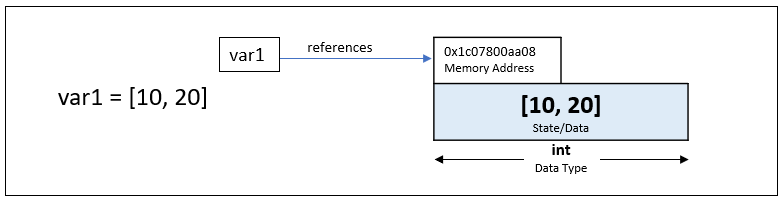
In the above example, there is only one variable referencing 0x20bfa819cc8
. So, the reference count of that object is 1. So, how do we check the reference count of the object, the variable is referencing. There are 2 ways to get the reference count of the object:
- Using getrefcount from sys module
In Python, by default, variables are passed by reference. Hence, when we run sys.getrefcount(var1) to get the reference count of var1, it creates another reference as to var1. So, keep in mind that it will always return one reference count extra. In this case, it will return 2. - Using c_long.from_address from ctypes module
In this method, we pass the memory address of the variable. So, ctypes.c_long.from_address(id(var1)) returns the value 1 as there is only one reference to the same object in the memory.
As you can see from the below code, getrefcount()
and from_address
returns reference count of 2 and 1 as expected.
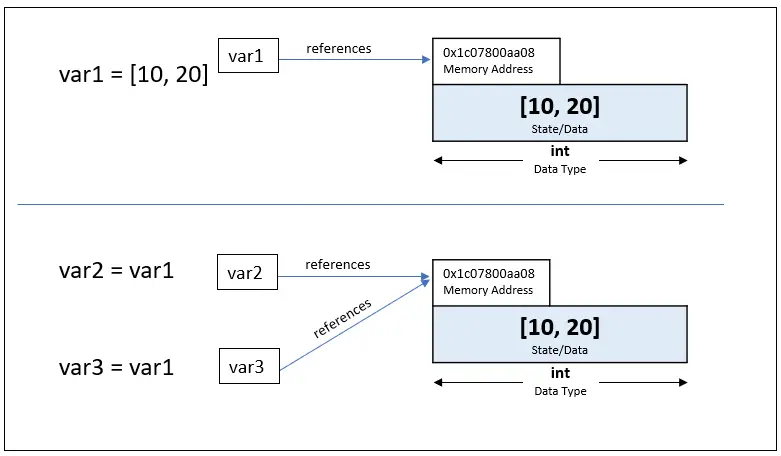
Let’s say if we execute var2 = var1, var3 = var1
, what will be the reference count of the object? You guessed it right!! It’s three as there are 3 variables referencing the same object in the memory.
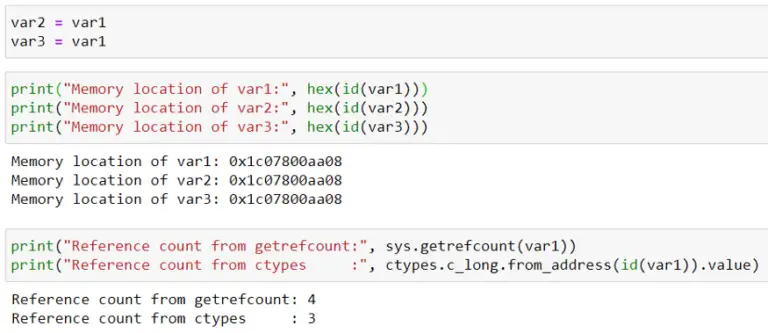
Once the variable var3 is set to None or if it gets deleted during the execution of the program, then the reference count reduces to 2 as you can see from the below code.

Similarly, when we set var2
to None or if it gets deleted during the execution of the program, then reference count reduces to 1 as you can see from the below code.

Finally, when var1
also goes away during the execution of the programs, reference count plays its role and release the memory to heap as there are no references to that object var1
was referring to earlier.
Conclusion
In this article, you have understood how reference counting works in Python. As mentioned earlier, as developers we don’t have to worry about this as the Python memory manager takes care of this behind the scenes. However, understanding how reference counting may help you when you are debugging memory leaks in Python.
Originally published at Medium on 23-Dec-2020.